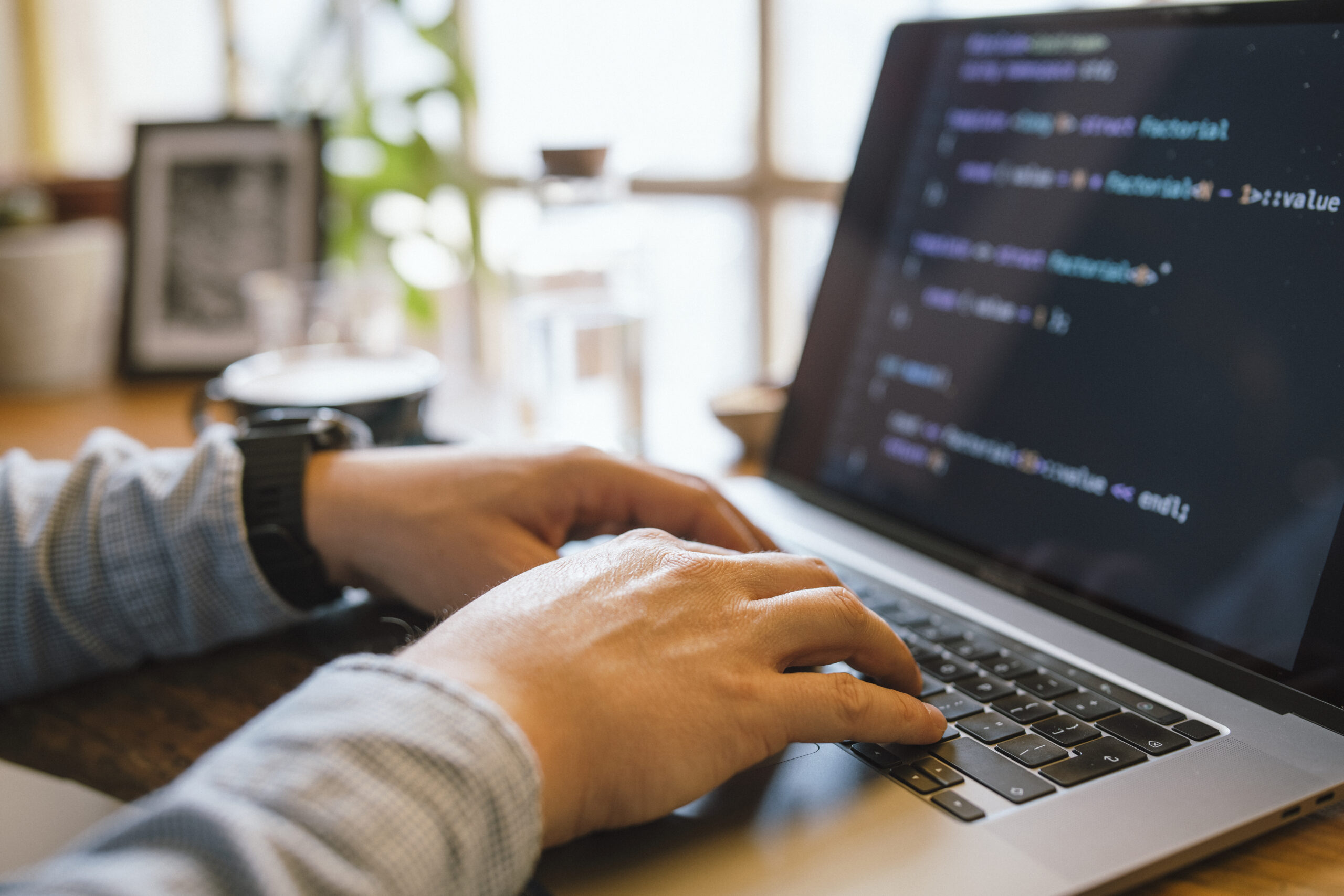
Debugging is one of the most vital — yet frequently disregarded — capabilities inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Completely wrong, and learning to think methodically to solve problems efficiently. Whether or not you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and radically help your efficiency. Here's various approaches to help you builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest means builders can elevate their debugging expertise is by mastering the resources they use every day. Though producing code is a person Component of growth, understanding how to connect with it properly throughout execution is equally important. Modern-day advancement environments come Outfitted with effective debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Choose, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code on the fly. When applied properly, they let you observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, such as Chrome DevTools, are indispensable for front-close builders. They let you inspect the DOM, observe network requests, see authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform disheartening UI concerns into workable duties.
For backend or procedure-level builders, applications like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle around operating processes and memory administration. Discovering these instruments may have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Edition Management devices like Git to understand code background, locate the exact instant bugs were being introduced, and isolate problematic changes.
In the end, mastering your resources implies heading further than default settings and shortcuts — it’s about developing an intimate knowledge of your improvement atmosphere in order that when difficulties crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you can spend resolving the particular challenge in lieu of fumbling by way of the method.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the issue. Ahead of jumping into the code or earning guesses, builders want to create a consistent atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of possibility, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a difficulty is gathering as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the much easier it turns into to isolate the exact disorders beneath which the bug takes place.
After you’ve gathered adequate information, seek to recreate the challenge in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical person interactions, or mimicking method states. If The problem seems intermittently, think about producing automated exams that replicate the edge circumstances or point out transitions involved. These exams not simply help expose the challenge but additionally avert regressions Down the road.
Occasionally, The problem may very well be atmosphere-distinct — it'd take place only on selected functioning methods, browsers, or beneath unique configurations. Applying tools like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It calls for endurance, observation, in addition to a methodical solution. But once you can regularly recreate the bug, you are presently midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools more successfully, check probable fixes safely and securely, and converse far more Plainly using your staff or end users. It turns an abstract criticism right into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Error Messages
Error messages are often the most valuable clues a developer has when something goes wrong. Rather than looking at them as frustrating interruptions, builders really should understand to treat mistake messages as immediate communications through the program. They typically let you know exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Start off by studying the information thoroughly and in comprehensive. Quite a few developers, specially when less than time strain, glance at the 1st line and right away start building assumptions. But deeper during the mistake stack or logs could lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — browse and recognize them first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a particular file and line selection? What module or operate brought on it? These concerns can guideline your investigation and stage you towards the responsible code.
It’s also practical to grasp the terminology from the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to acknowledge these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and up to date modifications during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, decrease debugging time, and become a a lot more economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers serious-time insights into how an software behaves, helping you understand what’s happening under the hood without having to pause execution or move in the code line by line.
A very good logging technique starts with understanding what to log and at what level. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and Deadly. Use DEBUG for in depth diagnostic details throughout improvement, INFO for typical gatherings (like prosperous start off-ups), Alert for prospective problems that don’t crack the appliance, ERROR for actual challenges, and Deadly when the program can’t carry on.
Avoid flooding your logs with abnormal or irrelevant info. A lot of logging can obscure important messages and decelerate your program. Focus on key situations, condition changes, enter/output values, and demanding conclusion factors in your code.
Structure your log messages Plainly and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in manufacturing environments wherever stepping via code isn’t doable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your applications, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a form of investigation. To efficiently establish and take care of bugs, developers should strategy the method similar to a detective resolving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Get started by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. Similar to a detective surveys a criminal offense scene, obtain just as much applicable information and facts as you can without leaping to conclusions. Use read more logs, exam conditions, and person stories to piece jointly a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes not too long ago been produced towards the codebase? Has this issue happened ahead of beneath comparable circumstances? The intention will be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a specific perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Pay back near attention to smaller specifics. Bugs often cover within the the very least anticipated destinations—like a missing semicolon, an off-by-one particular error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Momentary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, keep notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging procedure can preserve time for future concerns and enable Other people recognize your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method complications methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the best strategies to help your debugging skills and General growth effectiveness. Assessments don't just assistance catch bugs early but also serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint just the place and when a difficulty happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can quickly reveal regardless of whether a particular piece of logic is Operating as expected. Any time a exam fails, you straight away know wherever to glance, drastically minimizing enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after Formerly being preset.
Following, integrate integration checks and end-to-close assessments into your workflow. These aid make sure that many portions of your application work jointly easily. They’re particularly handy for catching bugs that take place in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to Consider critically about your code. To test a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and enjoy your test pass when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from a aggravating guessing activity into a structured and predictable method—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The difficulty from the new point of view.
When you are far too near to the code for way too very long, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue discover a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with improved tactics like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a fresh layer towards your skill set. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — but the payoff is huge. It can make you a far more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.